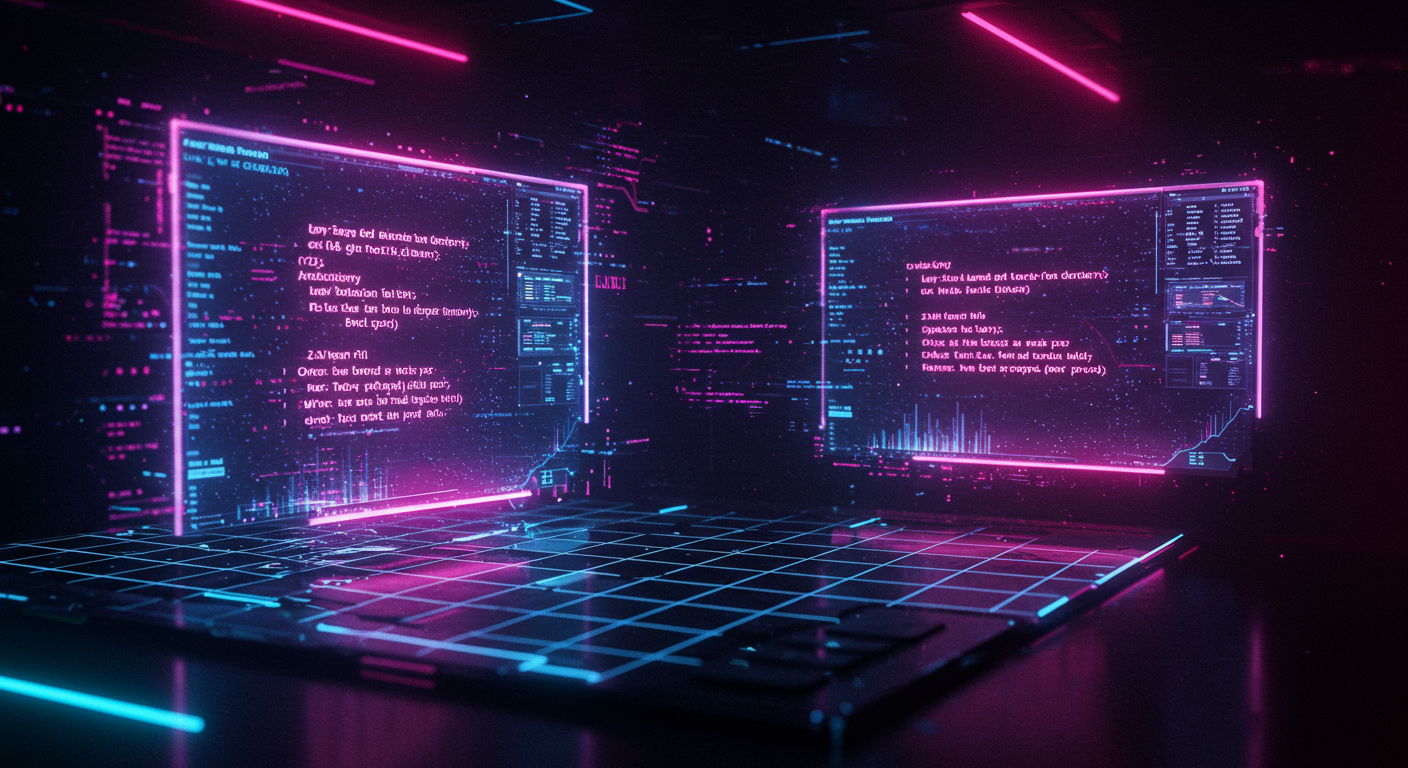
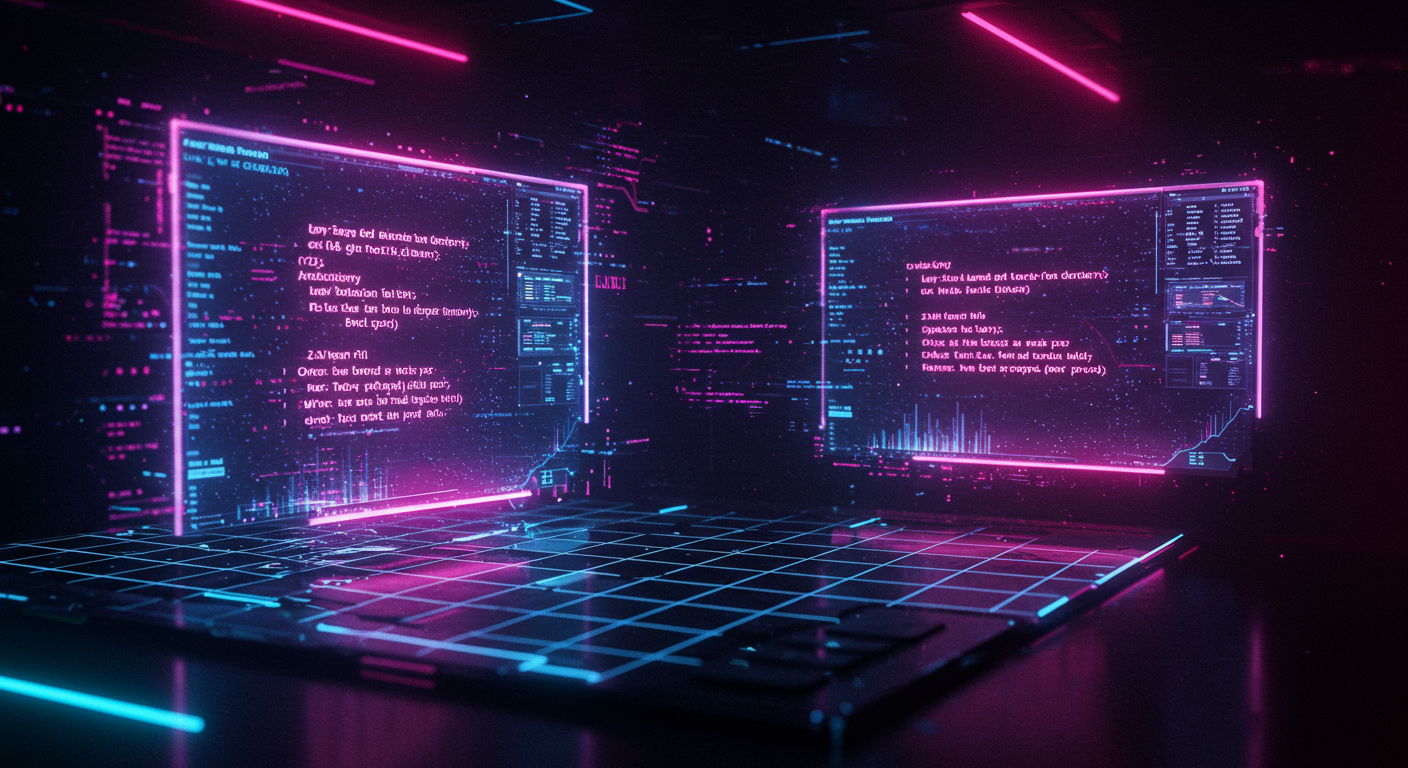
The Quick Tutorial on Python Lambda Functions That You Never Asked For
Let's be real, nobody wakes up in the morning thinking, "You know what I need more of in my life? Python lambda functions." But here we are. Buckle up, because this is the lambda tutorial you didn't ask for—but secretly need.
Lambda Functions: Anonymous, Lazy One-Liners
Lambda functions, also known as anonymous functions (because naming is overrated), let you squeeze a simple function into a single, no-nonsense line of code:
lambda arguments: expression
- Arguments: Stuff you feed the function.
- Expression: What the function spits out. And only one—don't get greedy.
For instance, incrementing a number by 1, because who has time for def
?
lambda x: x + 1
This is equivalent to:
def increment(x):
return x + 1
It's basically the Netflix of Python functions: fast, easy, and minimal commitment.
When Lambdas Actually Matter
Lambda functions are perfect when:
- You want a quick-and-dirty function without cluttering your namespace.
- Passing functions as arguments—especially with pandas'
apply()
.
Here's an actual example from real-world usage:
df['vector'] = df['content'].apply(lambda x: model.encode(x, normalize_embeddings=True))
Translation:
- Take each
content
item from your DataFrame. - Turn it into a vector (because vectors are cool).
- Do it without polluting your script with needless function names.
It's like hiring an intern for a five-minute task—without having to learn their name.
Lambda Goodness
- Concise: One-liner code beauty.
- Functional Programming Friendly: Easily passed around like a hot potato.
- Cleaner: Prevents namespace pollution, aka fewer things to regret later.
Lambda Badness
- Single Expression Limitation: Complex logic? Look elsewhere.
- Debugging Nightmare: Anonymous means nameless means frustration.
- Overuse Syndrome: Just because you can doesn't mean you should. (Looking at you, regex.)
Quick-and-Dirty Lambda Examples
Sorting a List
Here's where lambdas actually shine - complex sorting that would be a pain in the ass otherwise:
points = [(1, 2), (3, 1), (5, 0)]
sorted_points = sorted(points, key=lambda point: point[1])
print(sorted_points) # Output: [(5, 0), (3, 1), (1, 2)]
What's happening here? We're telling Python "sort these tuples, but ignore the first number and sort by the second one instead." The lambda grabs each tuple, extracts the second value (point[1]
), and sorted()
does the rest. Without lambdas, you'd need a whole separate function for this trivial operation. Bloody waste of code.
Filtering a List
When you need to separate the wheat from the chaff:
numbers = [1, 2, 3, 4, 5]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4]
The filter()
function is expecting another function that returns True
or False
. Our lambda takes each number, divides by 2, and checks if there's a remainder. No remainder? It's even, keep it. Otherwise, chuck it. The result is only the even numbers making it through the filter. It's like a bouncer at a club where odd numbers aren't cool enough to get in.
Transforming a List with Map
Need to apply the same operation to every item in a list? map()
+ lambda is your friend:
numbers = [1, 2, 3, 4]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16]
Here, map()
takes our lambda and applies it to each number in the list. The lambda just squares whatever it gets. So simple it's almost boring, but that's the point. You don't need a whole function definition ceremony for something this straightforward. It's the difference between sending a text and writing a formal letter – sometimes you just need to get the message across without the fluff.
Wrap-Up: Lambdas in a Nutshell
Lambda functions are your go-to for simple, anonymous tasks that aren't worth the full-blown function definition. Sure, they're limited. Yeah, debugging them is annoying. But when used wisely, they're elegant as hell.
So next time you need a function that's here for a good time—not a long time—reach for lambda.
And that's it. Tutorial over. Go forth and lambda responsibly (or don't—I'm not your boss).
Feature Image Prompt:
Generate an image. The aesthetic should be cyberpunk with colors of neon pink, blue and purple. Do not add any people. Create a futuristic digital scene featuring a sleek, neon-glowing computer interface that showcases snippets of Python lambda function code floating in holographic displays. Integrate abstract data streams, dynamic geometric grids, and circuit-like patterns that pulse with vibrant neon pink, blue, and purple hues to evoke the minimalist yet powerful nature of lambda functions in a high-tech, cyberpunk environment.